C++ and Programming Graphical User Interfaces Winter Term 2018/19
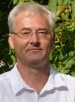
Prof. Dr.-Ing. habil.
Ralf Salomon
E-Mail
Tel.: +49 381 498 7260
Raum: 201
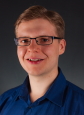
M.Sc.
Theo Gabloffsky
E-Mail
Tel.: +49 381 498 7255
Raum: 202
Organization
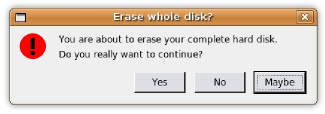
Lecturer-in-Charge: Ralf Salomon
Assistent: Theo Gabloffsky
Integrated Class: Tuesday: 11:15-17:00, Room 1219/1216, Building 1, Warnemünde
Description
Content
This module provides an introduction into object-oriented programming, the programming language C++, and the design and implementation of graphical user interfaces. These topics are covered in an integrated form in which the implementation of the graphical user interfaces serves as programming exercises to a large extent. In its current form, this module is a reformed combination of two previous modules, known as Einf¨hrung in die objektorientierte Programmierung and Programming Graphical User Interfaces.
The part on object-oriented programming includes the following concepts: classes, objects, method calls (messages), as well as public and private scope. These concepts are explained on an abstract level, and then applied in subsequent C++-exercises.
The part on graphical user interfaces (GUIs) includes their design, usability. The implementations will be done with Qt since it is platform independent (Windows, Linux, Unix, MacOS, etc.), entirely object oriented, coded in C++, and supports the programmer very well by providing very good (online) documentation as well as development tools. Furthermore, Qt provides quite sophisticated, high-level concepts such that just a few lines of C++ are sufficient to realize a first graphical user interface. In addition, Qt is freely available.
In the course of learning Qt and to get familiar with its concepts the students have to implement very small programs. Afterwards, the students are supposed to work on a decent robotics control task. Finally, the programs are presented to the class, and their usability are tested by a "dumb" test user. The last part is accompanied with an introduction on important object-oriented design concpets.
Goals
The two major goals of this class are: (1) the students are proficient in the design and realization of C++ programs, and (2) they are able to build graphical user interfaces that have a decent complexity. This is a good preparation for their further theses works.
Format
According to the module description, this class consists of two hours of lecturing as well as one hour of exercises each week. These six hours are organized in one row. Due to the nature of an integrated class, we switch between lectures and exercises on demand. The actual project work, which is devoted to the robot control task, is scheduled for January.
Prerequisites
Decent programming experiences in C. Without it chances for failing are high. If in doubt, please ask any of the staff members.
Exams
The exam will be oral (about 15 minutes) and will be by individual appointment. Please note that the successful accomplishment of the final programming project is required!
Vorlesungen als Video
Projektverwaltung mittels make und qmake
Signals und Slots in Qt
Scripts, Exercises and Samples
Tasks
1. Usability
2. A Notepad
2a. C-Struct Revisited
2b. Function Pointers
2c. Der Schwingkreis
2d. Der Schwingkreis in C++
2e. Operator and Method Overloading
3. Events
4. Call-Back Functions
5. Tutorial Part 1
6. Tutorial Part 2
7. Your Own Widgets
8. Timer Objects
9. The Make Tool
10. A Simple Dialog
10a. Namespaces
10b. Templates
10c. References
10d. Various Constructor Types
10e. Defining Operators
10f. Exception Handling in C++
10g. The C++ Standard Template Library
11. A Robot Application
Exercises and Samples
Manuals and Programming Frameworks
Literature
- Qt This web site, ordered by version, offers various tutorials, white papers, descriptions, tools, and an extensive documentation of all Qt classes.
- Qt 4 Einführung in die Applikationsentwicklung, Daniel Molkentin, Open Source Press, ISBN-13 978-3-937514-12-3. This book provides a very good introduction into Qt 4.
- Entwurfsmuster, Gamma et al., Addison-Wesley Verlag, ISBN 3-8273-1862-9.
- C++-Entwicklung mit Linux Thomas Wieland, Dpunkt Verlag, September 2002, ISBN 389864166X. This book provides a good introduction into C++.
- Die C++-Programmiersprache Bjarne Stroustrup. This book provides a good introduction into C++: "http://www.stroustrup.com/4th.html"; available in German and English in our library, see "http://katalog.ub.uni-rostock.de/CLK?IKT=4&TRM=The+C%2B%2B+programming+language".
- C++ Annotations Version Frank B. Brokken, University of Groningen. For knowledgeable users of C who make the transition to C++, ISBN 90 367 0470 7. Siehe auch: "https://fbb-git.github.io/cppannotations/cppannotations/html/index.html".
- C++- objektorientierte Programmierung von Anfang an, Helmut Erlenkötter, RoRoRo, ISBN 3-499 600 77-3.
- C++ GUI Programming with Qt4, Jasmin Blanchette and Mark Summerfield, Prentice Hall Open Source Software Development, ISBN-13: 978-0132354165. Siehe auch: "http://www.qtrac.eu/C++-GUI-Programming-with-Qt-4-1st-ed.zip" für eine kostenlose Download-Variante.
- Usability This web page provides a very good introduction into many relevant usability aspects.
- LinuxUser-11.03: This is a critical review of the usability of the KDE-project.
Class Schedule
Please note that because of the integrated nature of this class, the term Integrated Class includes both teacher-centered teaching and student-centered exercises on an on-demand basis. So, please do not expect a precise distinction between both.
Oktober: 16. 10. 2018: Lecture and Exercise/Laboratory
User Interfaces and Usability
- A brief history: command line, menues, graphical user interfaces
- Usability: what is it, how to measure it, design goals, design elements, walk through
- Working on Exercises 1 and 2
Oktober: 23. 10. 2018: Lecture and Exercise/Laboratory
From C to C++: A First Introduction
- Why C++?
- Comparison of C and C++?
- Object-oriented techniques in C: pseudo-virtual functions, function pointers, and attributes as structs
- Styles and paradigms in C++
- Technical terms and concepts: class, sub class, super class, attribute, operators, inheritance, messages, polymorphism
- Working on Exercises 2a and 2b
Oktober: 30. 10. 2018: Lecture and Exercise/Laboratory
Basic Programming Techniques in C++
- C++: types, literals, declaration, and initialization
- The C++ memory structure: stack, free store, static data and code segment
- C++-classes: from struct to class, objects, member, this-pointer, public and private, constructor, new, initialization list, single inheritance
- C++ language features
- C++ functions, function overloading, default arguments
- Working on Exercises 2c and 2d
November: 06. 11. 2018: Lecture and Exercise/Laboratory
The Design of a Graphical User Interface
- The X-windows system: hardware, drivers, X-server, X-clients, window managers, mouse, and keyboard
- Graphical elements: windows, and manipulations, such as zooming, resizing, and iconifying
- Events in window systems
- Working on Exercises 2e to 4
November: 13. 11. 2018: Lecture and Exercise/Laboratory
Graphical Systems and Introduction into Qt
- The design of the Qt library
- Events in Qt: Qt’s signals & slots concept
- First steps in Qt: the tutorials
- Qt’s implementation of signals & slots: the meta object compiler (MOC)
- Working on Exercises 5 and 6
November: 27. 11. 2018: Lecture and Exercise/Laboratory
Dialogs, Applications and The Make Tool
- Dialogs: how to implement, their internals, how to connect them with the application
- The main application window: the menue bar, tool bars, the central widget, the status bar, and action objects
- The Make Tool
- Working on Exercises 8-10
Dezember: 02. 12. 2018: Lecture and Exercise/Laboratory
Advanced Design and Implementation Techniques Part I
- Using declarations/directives
- Templates in C++
- Namespaces in C++
- Basics left overs: enumerations, const class members, input and output, references, and functions (call by value, call by reference, call by const-reference)
- Memory leaks
- Classes: destructor, constructors from one \ or more arguments, default constructor, copy constructor, copy assignment
- Working on Exercises 10a and 10b
Dezember: 11. 12. 2018: Lecture and Exercise/Laboratory
Advanced Design and Implementation Techniques Part II
- Operator overloading
- Abstract classes with protected/pure virtual functions
- Working on Exercises 10c and 10d
- Error Handling and the Standard Template Library
- Exceptions mittels try and catch
- Standard Template Library
- Generic programming and basic model: algorithms, containers, and iterators
- Predicates: functions, functions objects, lambdas, and inline code
- Automatic and range-based loops
Dezember: 18. 12. 2018: Lecture and Exercise/Laboratory
Error Handling and the Standard Template Library
- Exceptions mittels try and catch
- Standard Template Library
- Generic programming and basic model: algorithms, containers, and iterators
- Predicates: functions, functions objects, lambdas, and inline code
- Automatic and range-based loops
- Working on Exercises 10e to 10g
Januar: Exercise and Final Project Presentation
- Working on the final programming project: developing and testing various concepts
- Preparing for the project demonstration
- Final Project Presentation
Epuck Information
This page provides some information about the epuck's rfcomm interface and a set of source files. More information can be found at
Source Files
We will not program the firmware of the e-puck. Instead, we use the existing serial command interface. Just select program number 3, and turn on your epuck. The rest is done on the PC side.
- EPuck.zip provides a nearly complete implementation of the serial interface. This is the same file as the one on the GUI web page.
- github.com/awoody/e-puck a copy of the source code of the robot's firmware and some documentation.
Bluetooth
Connecting to the epuck is a pain. If you prefer different pain, switch to another operating system. The test program in EPuck.zip tries to work around many of the issues on linux.
Serial Commands
The epuck has a built-in serial-over-bluetooth interface. There are ascii and binary commands.
ASCII commands
Tabelle auf Epuck-Informationen (uni-rostock.de)
ASCII Kommandos
command | answer | meaning |
A | a,right,back,bottom | returns acceleration, values ? |
B,action | b | perform action on the green body LEDs; actions are 0=off, 1=on, 2=toggle |
C | c,position | returns the position of the mode switch; should be 3 |
D,left,right | d | sets the stepper motors' speed; values in steps per second; one step corresponds to 0.13 mm/s; min is about -1000, max is about 1000 |
E | e,left,right | returns the stepper motors' speed; values like D |
F,action | f | perform action on the front LED; values like B |
G | ? | infrared receiver ? |
H | much text | list of available commands |
I | 1,40,40,8,3200 | get the camera mode ( 40 by 40 pixels, 2 bytes each ) mode 1 = color (0 = grayscale), zoom 8 |
J,m,w,h,z,x1,y1 | ? | set the camera mode ? |
K | calibrating ... (and so on) < calibrate proximity sensors | |
L,number,action | 1 | perform action on one of the eigth LEDs; action values like B; numbers: 0 is front, increasing clockwise |
N | n,pr10,pr45,pr90,prb,plb,pl90,pl45,pl10 | read proximity sensors; values are 12 bit; 10, 45, 90 are degrees; b means back; l,r are left and right |
O | o,or10,or45,or90,orb,olb,ol90,ol45,ol10 | read ambient light sensors; values are 12 bit; 10, 45, 90 are degrees; b means back; l,r are left and right |
P,left,right | p | set the 16 bit step counters of the motors |
Q | q,left,right | get the 16 bit step counters of the motors |
R | r | wait some milliseconds, then reset the robot |
S | s | stop robot, switch of LEDsT,numbertplay sound sample |
U | u,mic1,mic2,mic3 | get the loudness at the three microphones |
V | v,version of sercom software | some text as C-string |
W? | ? | write I2C ? |
Y ? | ? | read I2C ? |
any other | z,Command not found |